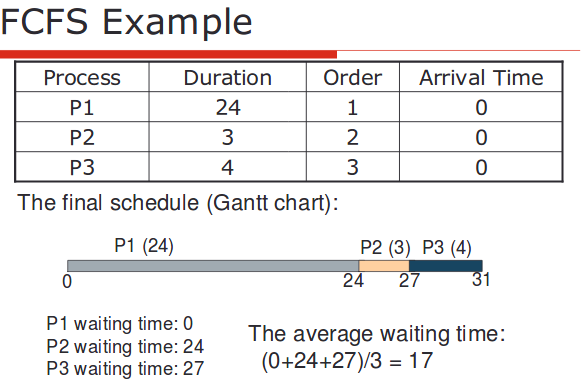
- Completion Time: Time at which process completes its execution.
- Turn Around Time: Time Difference between completion time and arrival time. Turn Around Time = Completion Time – Arrival Time
- Waiting Time(W.T): Time Difference between turn around time and burst time.
Waiting Time = Turn Around Time – Burst Time
In this post, we have assumed arrival times as 0, so turn around and completion times are same.
Implementation:
1- Input the processes along with their burst time (bt). 2- Find waiting time (wt) for all processes. 3- As first process that comes need not to wait so waiting time for process 1 will be 0 i.e. wt[0] = 0. 4- Find waiting time for all other processes i.e. for process i -> wt[i] = bt[i-1] + wt[i-1] . 5- Find turnaround time = waiting_time + burst_time for all processes. 6- Find average waiting time = total_waiting_time / no_of_processes. 7- Similarly, find average turnaround time = total_turn_around_time / no_of_processes.
// C++ program for implementation of FCFS
// scheduling with different arrival time
#include
using namespace std;
// Function to find the waiting time for all
// processes
void findWaitingTime(int processes[], int n, int bt[],
int wt[], int at[])
{
int service_time[n];
service_time[0] = 0;
wt[0] = 0;
// calculating waiting time
for (int i = 1; i < n ; i++)
{
// Add burst time of previous processes
service_time[i] = service_time[i-1] + bt[i-1];
// Find waiting time for current process =
// sum - at[i]
wt[i] = service_time[i] - at[i];
// If waiting time for a process is in negative
// that means it is already in the ready queue
// before CPU becomes idle so its waiting time is 0
if (wt[i] < 0)
wt[i] = 0;
}
}
// Function to calculate turn around time
void findTurnAroundTime(int processes[], int n, int bt[],
int wt[], int tat[])
{
// Calculating turnaround time by adding bt[i] + wt[i]
for (int i = 0; i < n ; i++)
tat[i] = bt[i] + wt[i];
}
// Function to calculate average waiting and turn-around
// times.
void findavgTime(int processes[], int n, int bt[], int at[])
{
int wt[n], tat[n];
// Function to find waiting time of all processes
findWaitingTime(processes, n, bt, wt, at);
// Function to find turn around time for all processes
findTurnAroundTime(processes, n, bt, wt, tat);
// Display processes along with all details
cout << "Processes " << " Burst Time " << " Arrival Time "
<< " Waiting Time " << " Turn-Around Time "
<< " Completion Time \n";
int total_wt = 0, total_tat = 0;
for (int i = 0 ; i < n ; i++)
{
total_wt = total_wt + wt[i];
total_tat = total_tat + tat[i];
int compl_time = tat[i] + at[i];
cout << " " << i+1 << "\t\t" << bt[i] << "\t\t"
<< at[i] << "\t\t" << wt[i] << "\t\t "
<< tat[i] << "\t\t " << compl_time << endl;
}
cout << "Average waiting time = "
<< (float)total_wt / (float)n;
cout << "\nAverage turn around time = "
<< (float)total_tat / (float)n;
}
// Driver code
int main()
{
// Process id's
int processes[] = {1, 2, 3};
int n = sizeof processes / sizeof processes[0];
// Burst time of all processes
int burst_time[] = {5, 9, 6};
// Arrival time of all processes
int arrival_time[] = {3, 3, 6};
findavgTime(processes, n, burst_time, arrival_time);
return 0;
}
Comments
Post a Comment